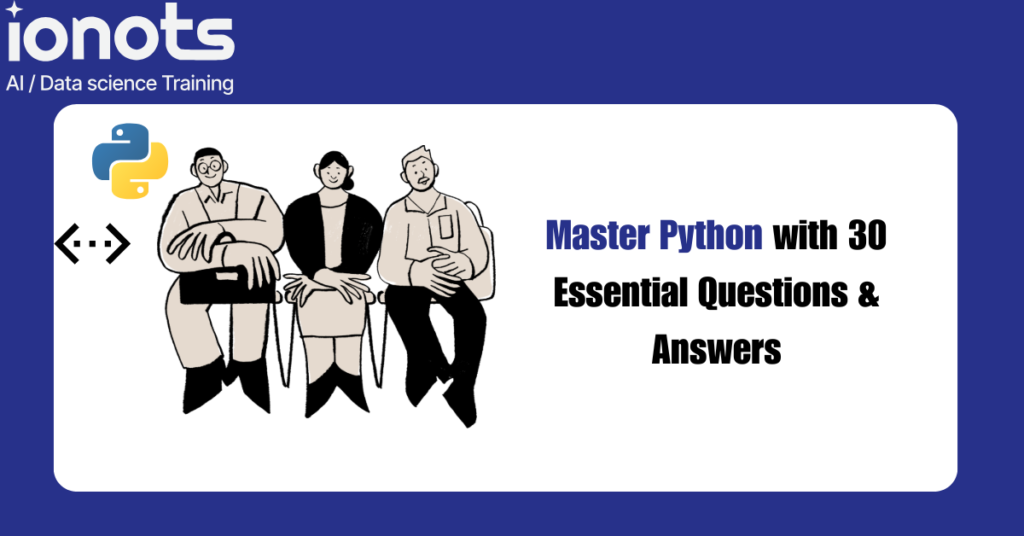
Introduction
Python is one of the most popular programming languages today, known for its simplicity, versatility, and vast ecosystem of libraries. Whether you are a beginner looking to get started or an experienced developer refining your skills, mastering Python involves both understanding theoretical concepts and hands-on coding.
In this blog, we cover 30 essential Python questions—ranging from fundamental concepts to advanced topics—ensuring you get a well-rounded grasp of the language.
Q1. Why is Python so popular?
Python is popular due to its simplicity, readability, and vast libraries that support diverse applications such as web development, data science, artificial intelligence, and automation.
Q2. What are the key features of Python?
Some key features of Python include:
- Easy to learn and use
- Interpreted language
- Dynamically typed
- Extensive libraries and frameworks
- Supports multiple programming paradigms (procedural, object-oriented, functional)
Q3. Write a Python program to print “Hello, World!”.
print("Hello, World!")
Q4. What is a variable in Python?
A variable in Python is a named reference to a value stored in memory. Unlike other languages, Python does not require explicit variable declaration.
x = 10
name = "Alice"
Q5. Explain mutable and immutable objects in Python.
- Mutable objects: Can be changed after creation (e.g., lists, dictionaries, sets).
- Immutable objects: Cannot be changed after creation (e.g., strings, tuples, integers).
Q6. What is a list in Python? Give an example.
A list is an ordered collection of elements that can store different data types.
my_list = [1, "apple", 3.5]
print(my_list[1]) # Output: apple
Q7. How is a tuple different from a list?
A tuple is similar to a list but immutable, meaning its elements cannot be changed after creation.
tuple_example = (1, 2, 3)
Q8. What is a dictionary in Python?
A dictionary stores data in key-value pairs.
dict_example = {"name": "Alice", "age": 25}
print(dict_example["name"]) # Output: Alice
Q9. Explain conditional statements in Python with an example.
Conditional statements help execute different code blocks based on conditions.
age = 18
if age >= 18:
print("Eligible to vote")
else:
print("Not eligible")
Q10. What is the difference between for and while loops in Python?
- For loop: Iterates over a sequence.
- While loop: Repeats as long as a condition is met.
for i in range(5):
print(i)
x = 0
while x < 5:
print(x)
x += 1
Q11. What are functions in Python? Provide an example.
Functions are reusable blocks of code.
def greet(name):
return "Hello, " + name
print(greet("Alice"))
Q12. What are common math functions in Python?
Python provides built-in math functions like abs()
, round()
, min()
, max()
.
print(abs(-10)) # Output: 10
Q13. List some common errors in Python.
- SyntaxError
- NameError
- TypeError
- IndexError
- KeyError
Q14. How do you pass a function as an argument?
def apply_func(func, value):
return func(value)
print(apply_func(abs, -5)) # Output: 5
Q15. What is list comprehension? Provide an example.
List comprehension provides a concise way to create lists.
squares = [x**2 for x in range(5)]
print(squares) # Output: [0, 1, 4, 9, 16]
Q16. Explain file handling in Python.
Python provides open()
for file handling.
with open("file.txt", "r") as file:
content = file.read()
Q17. What is debugging in Python?
Debugging is identifying and fixing errors in code using tools like print()
and the pdb
module.
Q18. What are classes and objects in Python?
Classes define objects, and objects are instances of classes.
class Car:
def __init__(self, brand):
self.brand = brand
car = Car("Toyota")
print(car.brand) # Output: Toyota
Q19. Explain lambda functions in Python.
Lambda functions are anonymous functions.
square = lambda x: x**2
print(square(4)) # Output: 16
Q20. What are regular expressions?
Regular expressions are used for pattern matching.
import re
match = re.search("hello", "hello world")
print(bool(match)) # Output: True
Q21. What is Python PIP?
PIP is a package manager for installing Python libraries.
pip install numpy
Q22. How do you read Excel files in Python?
import pandas as pd
df = pd.read_excel("data.xlsx")
Q23. What are iterators in Python?
Iterators are objects that can be iterated upon.
my_iter = iter([1, 2, 3])
print(next(my_iter)) # Output: 1
Q24. What is pickling in Python?
Pickling serializes objects for storage or transmission.
import pickle
data = {"name": "Alice"}
with open("data.pkl", "wb") as f:
pickle.dump(data, f)
Q25. How do you work with JSON in Python?
import json
data = '{"name": "Alice"}'
parsed = json.loads(data)
print(parsed["name"]) # Output: Alice
Q26. How do you handle exceptions in Python?
Using try-except blocks.
try:
print(1 / 0)
except ZeroDivisionError:
print("Cannot divide by zero")
Q27. What is the difference between shallow and deep copy?
- Shallow copy: Copies references.
- Deep copy: Creates independent copies.
import copy
original = [[1, 2], [3, 4]]
shallow = copy.copy(original)
deep = copy.deepcopy(original)
Q28. What are decorators in Python?
Decorators modify functions.
def decorator(func):
def wrapper():
print("Before function execution")
func()
print("After function execution")
return wrapper
@decorator
def say_hello():
print("Hello!")
say_hello()
Q29. How does Python handle memory management?
Python uses reference counting and garbage collection.
Q30. What are Python generators?
Generators yield values lazily.
def gen():
yield 1
yield 2
print(list(gen())) # Output: [1, 2]
Conclusion
Mastering Python requires both theoretical knowledge and practical application. By exploring these 30 questions, you will have a solid understanding of fundamental and advanced concepts in Python. Keep practicing, and happy coding!
The official Python website emphasizes that the best way to learn Python is by diving in and getting hands-on. Theory is essential, but practical coding experience solidifies your understanding. This blog follows that philosophy—covering both fundamental concepts and hands-on coding challenges to help you master Python efficiently. So, roll up your sleeves and start coding!
Related Blog Posts
Don’t forget to check out our other detailed guides:
- Power BI vs. Tableau: Which to Learn in 2025?
- SQL Essentials for Data Analysis
- NumPy: Your Ultimate Guide to Numerical Computing
- Pandas: Master Data Manipulation in Python
- Scikit-learn: Machine Learning Made Simple
- Matplotlib Made Easy: Key Tips for Visualizing Data
- Unlock Insights: Matplotlib, Seaborn & Dash Mastery
Consequently, if you’re eager to take your skills to the next level, our specialized courses offer comprehensive training in:
- Advanced NumPy techniques
- Data manipulation
- Machine learning fundamentals
- AI and deep learning concepts
Explore Our Data Science and AI Career Transformation Course